A simple frontend workflow for Gulp
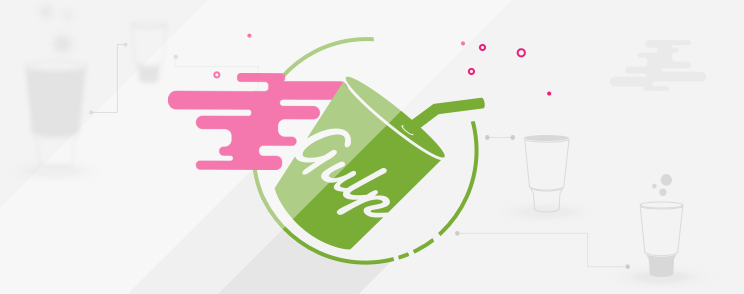
What is Gulp?
Gulp is a task/build runner which uses Node.js for web development. In this tutorial, I will not explain how to create your Gulp plugin but I will show you how you can create a simple, basic, customized build process for your frontend workflow to ease your job.
Gulp is often used for automated front end tasks like:
- Compiling preprocessors like Sass or Less
- Optimizing assets like images, CSS and Javascripts
- Including browsers prefixes with Autoprefixer
- Injecting CSS in browser without reloading page whenever file is saved
- Spinning up a web server
This is not, of course, a comprehensive list of things Gulp can do. There are thousands of plugins which you can use or you can build your own.
Step 1: Install Node.js
Because Gulp is built on Node.js (Javascript runtime built on Chrome’s V8 Javascript engine), first we need to install Node.js.
Node.js can be downloaded for Windows, Mac and Linux at nodejs.org/download/. You can also install it from the command line using the package manager.
Once installed, open a command prompt and enter:
$ node-v
This will show a Node.js version number if it is a correctly installed.
Step 2: Install Gulp
Now we can install Gulp using by using the following command in command line:
$ sudo npm install gulp -g
Note: Mac users need the sudo keyword. “$” in the code above just symbolize the command prompt. You don’t need to enter it.
The “-g” option in the command line tells to install Gulp globally, not just for the specific project. It allows you to use Gulp command anywhere on your system.
Now, we can make a project that uses Gulp.
Step 3: Set Up a Gulp Project
Go to
root/skin/frontend/design_package/default/
In this folder, we will create a Gulp config file and install all Gulps dependencies. Enter the command below in command line:
$ npm init
The “npm init” command creates a package.json file in which are stored information like dependencies, project name etc.
Once the package.json file is created, we can install Gulp and other plugins used in this tutorial.
Step 4: Installing Gulp
Install Gulp locally in the same folder. This will create “node_modules” folder where are all our dependencies files located. Enter the command in command line:
$ npm install gulp --save-dev
Great, now we can move on to install Gulp-Sass plugin.
Step 5: Installing Gulp-sass
Gulp Sass is a libsass version of Gulp. Libsass is a much faster in compiling sass files than Ruby.
Enter the command in command line:
$ npm install gulp-sass --save-dev
Great, move on to the next dependency, Gulp Source Maps.
Step 6: Install Gulp Source Maps
Gulp Source Maps is useful for debugging. You can see in which sass file are styles located for the inspected element in the browsers Inspector tool.
Enter the command in command line:
$ npm install gulp-sourcemaps --save-dev
Step 7: Install Autoprefixer
Autoprefixer for Gulp helps us to write CSS without vendor prefixes. It will automatically search if there is a need to add vendor prefix for some newly CSS properties. Autoprefixer is pulling data from caniuse.com site and adds prefixes if there is a need for it.
In the command line tool, enter command:
$ npm install gulp-autoprefixer --save-dev
Step 8: Install Browser Sync
BrowserSync is one of my favorites. It can reload a browser page after file saving, inject CSS without reloading page, sync browser page screen and your browsing actions through devices. Very handy.
Write in the command line:
$ npm install browser-sync --save-dev
Step 9: Create a Gulp config file
Create a new file gulpfile.js in the same folder. This will be the main configuration file for the Gulp where we write our tasks.
Open up gulpfile.js and write:
var gulp = require('gulp');
var sass = require('gulp-sass');
var sourcemaps = require('gulp-sourcemaps');
var autoprefixer = require('gulp-autoprefixer');
var browserSync = require('browser-sync').create();
We need to require all dependencies we have installed. The requirement statement tells Node to look into the node_modules folder for a package. Once the package is found, we assign its contents to the variable.
Next step is to create two variables used by GulpSass plugin for compiling sass files and we will assign to them a path for our Sass files and path for our compiled CSS file.
Write:
var input = './scss/**/*.scss';
var output = './css';
We can now begin to write a Gulp sass task. Add a code below:
gulp.task('sass', function () {
return gulp.src(input)
.pipe(sourcemaps.init())
.pipe(sass(errLogToConsole: true,outputStyle: 'compressed'))
.pipe(autoprefixer(browsers: ['last 2 versions', '> 5%', 'Firefox ESR']))
.pipe(sourcemaps.write())
.pipe(gulp.dest(output))
.pipe(browserSync.stream());
});
Add a watcher task for watching Scss file changes and BrowserSync settings. Add a code below:
// Watch files for change and set Browser Sync
gulp.task('watch', function() {
// BrowserSync settings
browserSync.init({
proxy: "mydomain.loc",
files: "./css/styles.css"
});
// Scss file watcher
gulp.watch(input, ['sass'])
.on('change', function(event){
console.log('File' + event.path + ' was ' + event.type + ', running tasks...')
});
});
Finally we create our default/main task which will run all above task. Add a code below:
// Default task
gulp.task('default', ['sass', 'watch']);
Our final gulpfile.js should look like this:
var gulp = require('gulp');
var sass = require('gulp-sass');
var sourcemaps = require('gulp-sourcemaps');
var autoprefixer = require('gulp-autoprefixer');
var browserSync = require('browser-sync').create();
var input = './scss/**/*.scss';
var output = './css';
gulp.task('sass', function () {
return gulp.src(input)
.pipe(sourcemaps.init())
.pipe(sass(errLogToConsole: true,outputStyle: 'compressed'))
.pipe(autoprefixer(browsers: ['last 2 versions', '> 5%', 'Firefox ESR']))
.pipe(sourcemaps.write())
.pipe(gulp.dest(output))
.pipe(browserSync.stream());
});
// Watch files for change and set Browser Sync
gulp.task('watch', function() {
// BrowserSync settings
browserSync.init({
proxy: "mydomain.loc",
files: "./css/styles.css"
});
// Scss file watcher
gulp.watch(input, ['sass'])
.on('change', function(event){
console.log('File' + event.path + ' was ' + event.type + ', running tasks...')
});
});
// Default task
gulp.task('default', ['sass', 'watch']);
To run above tasks, enter
$ gulp default
command in the command line.
Hope this will help someone to automate frontend workflow with Gulp. Thanks for reading!
8 comments
If you are looking for something that stays with core magento style and structures, our team has developed this magento-2-gulp that supports:
default magento 2 project structure with theme config inside dev/tools/grunt/configs/themes.js
installation with composer
.less
browsersync and liverreload
compiling es6+ with Babel
creating svg sprites
Hi Vanja,
Nice article thank you!
You miss object {} on theses lines:
.pipe(sass
.pipe(autoprefixer
I am using NPM Scripts as a workflow: https://github.com/tpkahlon/npm-app.
Hi that’s cool. But if don’t want write a lot of different gulp tasks try this – https://github.com/wwwebman/gulp-webpack-starter. This based on gulp task and Webpack middlewares for js bundling.
Maybe will be helpful for someone.
Hey Vanja,
It’s a great article thank you,
Just I found a small typo in your final gulpfile , you forget {} in your objet .
Hello Vanja,
I wonder if you ever had to work with some task-runner with multiple themes and only one task-runner file.
Hi Vanja,
Could you please update Step 8? It should be “npm install -g browser-sync” not the “autosync”
Thanks for noticing! It’s corrected!