Magento is a great eCommerce platform, it comes with so many built in features some of which you will probably never use. However one feature that we hear a lot of complain about is the lack of possibility to have a Shopping Cart Promotion Rule that targets the products custom options. If you login to Magento admin, and go to Promotions > Shopping Cart Price Rules > Add New Rule, then click on the Conditions tab of the Shopping Cart Price Rule edit screen you will see a screen like the one shown below.
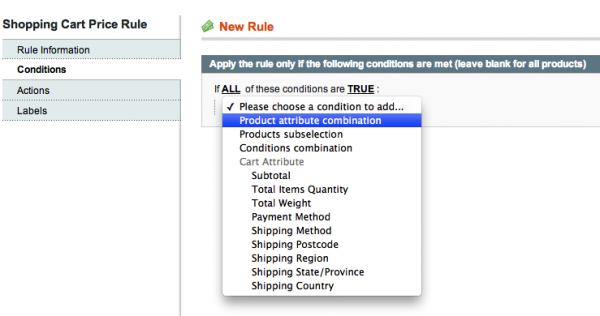
As you can see on that screen, there is no mention of products custom options, only its attributes.
In this article I’m gonna show you a nice little, cheap trick on how you can achieve the effect of having Shopping Cart Promotion Rule for Product with Custom Options. It will require a few lines of code to add and a little tiny bit of “manual calculation”. We will extract the code into our special little Inchoo_QuoteItemRule extension. Before we start writing the code, lets first explain what “Quote” has to do with “Custom Options”. Imagine we have a product product with SKU assigned “test_no2”. That product has two custom options (Color & size) and every option value has its SKU assigned as well like those shown on the image below.
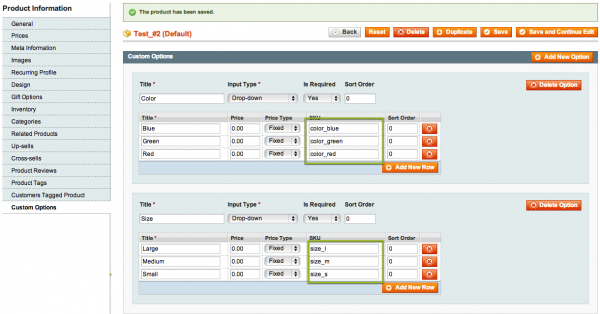
Now if we add that product to cart a product with selected Green color and Medium size option then this will get recorded under sales_flat_quote_item database table as entry with SKU value of “test_no2-color_green-size_m“, which is actualy and entry with the following formula:
product main SKU + “-” + first custom option SKU + “-” + second custom option SKU + “-” + … nth custom option SKU.
So the idea is that we actually create a rule condition that would allow us to have a Cart Item Attribute condition, like shown on image below, since Cart Item Attribute is actually a sales_flat_quote_item table entry field to put it like that. And we said that adding a product with custom option to cart results in sales_flat_quote_item table entry with SKU value of “test_no2-color_green-size_m“.
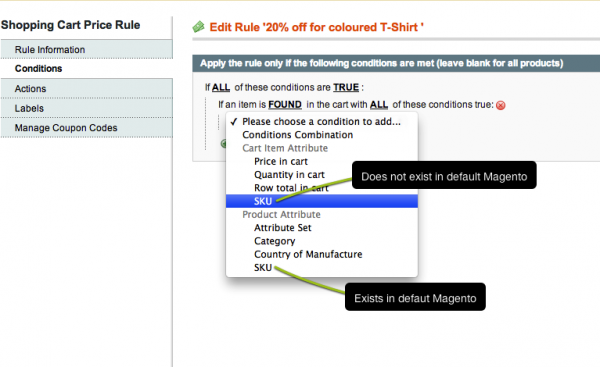
Now lets jump into the code, we will need 3 files to achieve the desired functionality.
app/etc/modules/Inchoo_QuoteItemRule.xml:
<?xml version="1.0"?>
<config>
<modules>
<Inchoo_QuoteItemRule>
<active>true</active>
<codePool>community</codePool>
</Inchoo_QuoteItemRule>
</modules>
</config>
app/code/community/Inchoo/QuoteItemRule/etc/config.xml:
<?xml version="1.0"?>
<config>
<modules>
<Inchoo_QuoteItemRule>
<version>1.0.0.0</version>
</Inchoo_QuoteItemRule>
</modules>
<global>
<models>
<salesrule>
<rewrite>
<rule_condition_product>Inchoo_QuoteItemRule_Model_SalesRule_Rule_Condition_Product</rule_condition_product>
</rewrite>
</salesrule>
</models>
</global>
</config>
app/code/community/Inchoo/QuoteItemRule/Model/SalesRule/Rule/Condition/Product.php:
<?php
class Inchoo_QuoteItemRule_Model_SalesRule_Rule_Condition_Product extends Mage_Rule_Model_Condition_Product_Abstract
{
protected function _addSpecialAttributes(array &$attributes)
{
parent::_addSpecialAttributes($attributes);
$attributes['quote_item_qty'] = Mage::helper('salesrule')->__('Quantity in cart');
$attributes['quote_item_price'] = Mage::helper('salesrule')->__('Price in cart');
$attributes['quote_item_row_total'] = Mage::helper('salesrule')->__('Row total in cart');
/* @inchoo */
$attributes['quote_item_sku'] = Mage::helper('salesrule')->__('SKU');
/* @inchoo */
}
public function validate(Varien_Object $object)
{
$product = false;
if ($object->getProduct() instanceof Mage_Catalog_Model_Product) {
$product = $object->getProduct();
} else {
$product = Mage::getModel('catalog/product')
->load($object->getProductId());
}
$product
->setQuoteItemQty($object->getQty())
->setQuoteItemPrice($object->getPrice())
->setQuoteItemRowTotal($object->getBaseRowTotal())
/* @inchoo */
->setQuoteItemSku($object->getSku())
/* @inchoo */;
return parent::validate($product);
}
}
We basically just needed to add two lines of code for the whole thing to work (you can see those two lines surrounded by “/* @inchoo */” comment). Now if you revisit the Promotions > Shopping Cart Price Rules > Add New Rule, then click on the Conditions tab, select the Product Attribute Combination as first condition then under the next condition dropdown you will see SKU under Cart Item Attribute like shown on the screenshot above. So finally, we can create a Shopping Cart Promotion Rule for Product with Custom Options like shown on the images below.
Hope it helps.
P.S. This example was provided in its simplest form, mostly just to demonstrate a quick “fix” for product custom options rule. More robust solution would be more proper for serious projects.